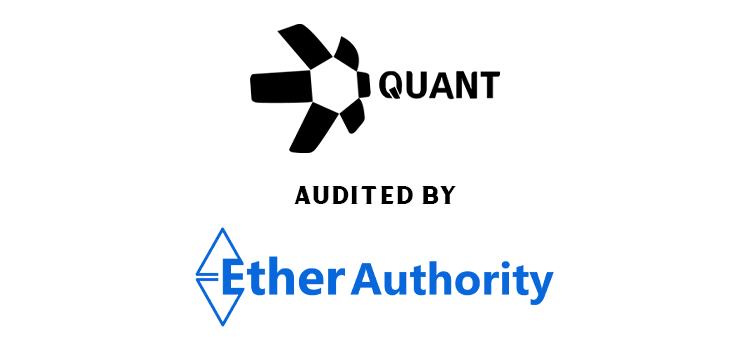
Project Background
- This Solidity contract implements a standard ERC20 token with additional functionality for a crowdsale. Let’s break it down:
- ERC20Basic:
- Interface defining essential ERC20 functions: `totalSupply()`, `balanceOf()`, and `transfer()`.
- It emits a `Transfer` event upon successful token transfer.
- SafeMath Library:
- A library providing arithmetic functions with safety checks to prevent overflow and underflow.
- BasicToken:
- Inherits from ERC20Basic and uses SafeMath library.
- Implements the `totalSupply()` and `balanceOf()` functions.
- Implements the `transfer()` function with safety checks for valid addresses and sufficient balance.
- The total supply is initialized to a fixed value of 45,467,000 QNT tokens.
- ERC20 Interface:
- An interface extending ERC20Basic with additional functions: `allowance()`, `transferFrom()`, and `approve()`.
- It emits an `Approval` event upon successful approval.
- StandardToken:
- Inherits from ERC20 and BasicToken.
- Implements additional functionalities such as `approve()`, `allowance()`, `transferFrom()`, `increaseApproval()`, and `decreaseApproval()`.
- Defines constants for token name, symbol, and decimals.
- Defines a `crowdsale` address and a modifier `onlyCrowdsale` to restrict certain functions to the crowdsale contract.
- Implements a `mint()` function that allows the crowdsale contract to mint tokens to specific addresses.
- Overrides the `transferFrom()` function with additional safety checks for valid addresses, sufficient balance, and allowance.
- Implements the `approve()` function to allow the spender to spend the specified amount on behalf of the owner.
- Implements `increaseApproval()` and `decreaseApproval()` functions to atomically increase or decrease the allowance.
- Overall, this contract provides a standard ERC20 token functionality with additional features such as minting and allowance manipulation, along with safety checks to prevent common vulnerabilities like overflow and underflow.
Website: quant.network
Executive Audit Summary
- According to the standard audit assessment, the Customer`s solidity smart contracts are “Secured”. This token contract does not have any ownership control, hence it is 100% decentralized.
- We used various tools like Slither, Solhint and Remix IDE. At the same time this finding is based on critical analysis of the manual audit.
- We found 0 critical, 0 high, 0 medium, 0 low and 7 very low-level issues.
Audit Report in PDF
Audit Report Flip book
Please wait while flipbook is loading. For more related info, FAQs and issues please refer to DearFlip WordPress Flipbook Plugin Help documentation.