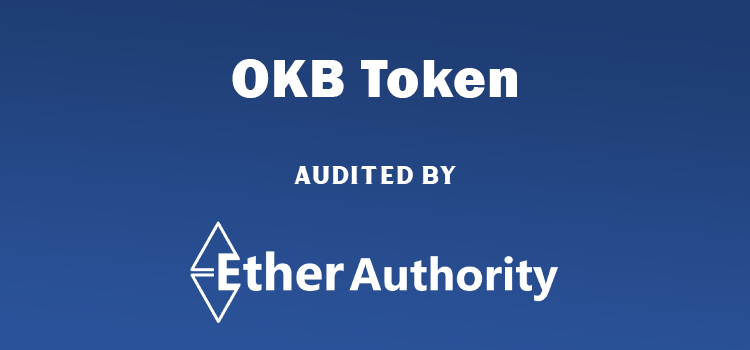
Project Background
- This Solidity contract seems to be an implementation of a token contract for OKB (the cryptocurrency of OKEx exchange) with functionalities like transferring tokens, pausing/unpausing transfers, approving transfers, managing allowances, freezing/unfreezing addresses, and adjusting token supply.
- Here’s a breakdown of the key components and functionalities:
- SafeMath Library: This library is used for arithmetic operations to prevent overflows and underflows.
- Contract Variables:
- balances: Mapping to store the balance of each address.
- totalSupply: Total supply of the token.
- name, symbol, decimals: Metadata of the token.
- _allowed: Mapping to track allowances for each address.
- owner: Address of the contract owner.
- paused: Boolean flag to control whether transfers are paused or not.
- lawEnforcementRole: Address with special permissions.
- frozen: Mapping to track frozen addresses.
- supplyController: Address controlling token supply.
- Events: Events are used to log significant contract actions.
- Modifiers:
- onlyOwner: Restricts functions to be called only by the contract owner.
- whenNotPaused: Restricts functions to be called only when transfers are not paused.
- onlyLawEnforcementRole: Restricts functions to be called only by the law enforcement role.
- onlySupplyController: Restricts functions to be called only by the supply controller.
- Functions:
- initialize(): Initializes the contract.
- totalSupply(), balanceOf(), allowance(): Getters for total supply, balance of an address, and allowance of spender.
- transfer(), transferFrom(): Transfers tokens from one address to another.
- approve(), increaseAllowance(), decreaseAllowance(): Approves spender to spend tokens on behalf of the owner.
- pause(), unpause(): Pauses/unpauses transfers.
- setLawEnforcementRole(), wipeFrozenAddress(), freeze(), unfreeze(): Manage frozen addresses by the law enforcement role.
- setSupplyController(), increaseSupply(), decreaseSupply(): Manage token supply.
- The OKBImplementation contract is a Pausable ERC20 token with Burn and Mint controlled by a central supply controller.
- This contract also includes external methods for setting a new implementation contract for the proxy.
- OKB Token smart contracts offer various functions like pause/unpause contracts and freeze/unfreeze address balances for transferring.
- Overall, this contract provides functionalities for managing a token ecosystem including ownership, transfers, allowances, freezing addresses, and controlling token supply, with certain permissions delegated to specific roles.
Website: okx.com
Executive Audit Summary
- According to the standard audit assessment, the Customer`s solidity smart contracts are “Poor Secured” Also, these contracts contain owner control, which does not make them fully decentralized.
- We used various tools like Slither, Solhint and Remix IDE. At the same time this finding is based on critical analysis of the manual audit.
- We found 1 critical, 0 high, 0 medium, 1 low and 9 very low-level issues.
Audit Report in PDF
Audit Report Flip book
Please wait while flipbook is loading. For more related info, FAQs and issues please refer to DearFlip WordPress Flipbook Plugin Help documentation.